Search Results
Compute the integrand: matrix element, PDFs, jacobians. More...
Detailed Description
Compute the integrand: matrix element, PDFs, jacobians.
Summary
Evaluate the matrix element, parton density functions, jacobians, phase-space density terms, flux factor, ..., to define the final quantity to be integrated.
The matrix element has to be evaluated on all the initial and final particles' 4-momenta. In most cases, a subset of those particles are given by a Block. Blocks produce several equivalent solutions for those particles, and the matrix element, ..., has to be computed on each of those solutions, along with the rest of the particles in the event. For that, you need to use a Looper module, with this module in the execution path.
To define the integrand, the quantities produced by this module (one quantity per solution) has to be summed. Use the DoubleSummer module for this purpose.
In more details
In the following, the set of solutions will be indexed by \(j\). Particles produced by the Block will be called 'invisible', while other, uniquely defined particles in the event will be called 'visible'. Since initial state momenta are computed from the whole event, they have the same multiplicity as the 'invisibles' and will therefore also be indexed by \(j\).
- Warning
- Keep in mind that the loop describe below is not done by this module. A Looper must be used for this.
If no invisibles are present and the matrix element only has to be evaluated on the uniquely defined visible particles, this module can still be used to define the integrand: no loop is done in this case, and the index \(j\) can be omitted in the following.
As stated above, for each solution \(j\), this modules's ouput is a scalar \(I\):
\[ I_j = \frac{1}{2 x_1^j x_2^j s} \times \left( \sum_{i_1, i_2} \, f(i_1, x_1^j, Q_f^2) \, f(i_2, x_2^j, Q_f^2) \, \left| \mathcal{M}(i_1, i_2, j) \right|^2 \right) \times \left( \prod_{i} \mathcal{J}_i \right) \]
where:
- \(s\) is the hadronic centre-of-mass energy.
- \(\mathcal{J}_i\) are the jacobians.
- \(x_1^j, x_2^j\) are the initial particles' Björken fractions (computed from the entry \(j\) of the initial states given as input).
- \(f(i, x^j, Q_f^2)\) is the PDF of parton flavour \(i\) evaluated on the initial particles' Björken- \(x\) and using factorisation scale \(Q_f\).
- \(\left| \mathcal{M}(i_1, i_2, j) \right|^2\) is the matrix element squared evaluated on all the particles' momenta in the event, for solution \(j\). Along with the PDFs, a sum is done over all the initial parton flavours \(i_1, i_2\) defined by the matrix element.
Expected parameter sets
Some inputs expected by this module are not simple parameters, but sets of parameters and input tags. These are used for:
- matrix element:
card
(string): Path to the the matrix element'sparam_card.dat
file.
- particles:
inputs
(vector(LorentzVector)): Set of particles.ids
: Parameter set used to link the visibles to the matrix element (see below).
Linking inputs and matrix element
The matrix element expects the final-state particles' 4-momenta to be given in a certain order, but it is agnostic as to how the particles are ordered in MoMEMta. It is therefore necessary to specify the index of each input (visible and invisible) particle in the matrix element call. Furthermore, since the matrix element library might define several final states, each input particle's PDG ID has to be set by the user, to ensure the correct final state is retrieved when evaluating the matrix element.
To find out the ordering the matrix element expects, it is currently necessary to dig into the matrix element's code. For instance, for the fully leptonic \(t\overline{t}\) example shipped with MoMEMta, the ordering and PDG IDs can be read from here.
In the Lua configuation for this module, the ordering is defined through the ids
parameter set mentioned above. For instance,
particles = {
inputs = { 'input::particles/1', 'input::particles/2' },
ids = {
{
me_index = 2,
pdg_id = 11
},
{
me_index = 1,
pdg_id = -11
}
}
}
means that the particle vector corresponds to (electron, positron), while the matrix element expects to be given first the positron, then the electron.
Integration dimension
This module requires 0 phase-space point.
Global Parameters
Name | Type | Description |
---|---|---|
energy | double | Hadronic centre-of-mass energy (GeV). |
Parameters
Name | Type | Description |
---|---|---|
use_pdf | bool, default true | Evaluate PDFs and use them in the integrand. |
pdf | string | Name of the LHAPDF set to be used (see full list). |
pdf_scale | double | Factorisation scale used when evaluating the PDFs. |
matrix_element | string | Name of the matrix element to be used. |
matrix_element_parameters | ParameterSet | Set of parameters passed to the matrix element (see above explanation). |
override_parameters | ParameterSet (optional) | Overrides the value of the ME parameters (usually those specified in the param card) by the ones specified. |
Inputs
Name | Type | Description |
---|---|---|
initialState | vector(vector(LorentzVector)) | Sets of initial parton 4-momenta (one pair per invisibles' solution), typically coming from a BuildInitialState module. |
particles | ParameterSet | Set of parameters defining the particles (see above explanation). |
jacobians | vector(double) | All jacobians defined in the integration (transfer functions, generators, blocks...). |
Outputs
Name | Type | Description |
---|---|---|
integrands | vector(double) | Vector of integrands (one per invisibles' solution). All entries in this vector will be summed by MoMEMta to define the final integrand used by Cuba to compute the integral. |
Definition at line 150 of file MatrixElement.cc.
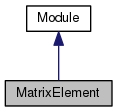
Public Member Functions | |
MatrixElement (PoolPtr pool, const ParameterSet ¶meters) | |
virtual void | beginIntegration () |
Called once at the beginning of the integration. | |
virtual Status | work () override |
Main function. More... | |
![]() | |
Module (PoolPtr pool, const std::string &name) | |
Constructor. More... | |
virtual void | configure () |
Called once at the beginning of the job. | |
virtual void | beginPoint () |
Called once when a new PS point is started. More... | |
virtual void | beginLoop () |
Called once at the beginning of a loop. More... | |
virtual void | endLoop () |
Called once at the end of a loop. More... | |
virtual void | endPoint () |
Called once when a PS point is finished. More... | |
virtual void | endIntegration () |
Called once at the end of the integration. | |
virtual void | finish () |
Called once at the end of the job. | |
virtual std::string | name () const final |
Additional Inherited Members | |
![]() | |
enum | Status : std::int8_t { OK, NEXT, ABORT } |
![]() | |
static std::string | statusToString (const Status &status) |
static bool | is_virtual_module (const std::string &name) |
Test if a given name correspond to a virtual module. More... | |
![]() | |
template<typename T , typename... Args> | |
std::shared_ptr< T > | produce (const std::string &name, Args... args) |
Add a new output to the module. More... | |
template<typename T > | |
Value< T > | get (const std::string &module, const std::string &name) |
template<typename T > | |
Value< T > | get (const InputTag &tag) |
![]() | |
PoolPtr | m_pool |
Member Function Documentation
◆ work()
|
inlineoverridevirtual |
Main function.
This method is called for each integration step. The module's logic and work happen here.
You'll usually want to override this function if you want your module to perform some task.
Reimplemented from Module.
Definition at line 242 of file MatrixElement.cc.
The documentation for this class was generated from the following file:
- modules/MatrixElement.cc