Parent class for all the modules. More...
#include <Module.h>
Detailed Description
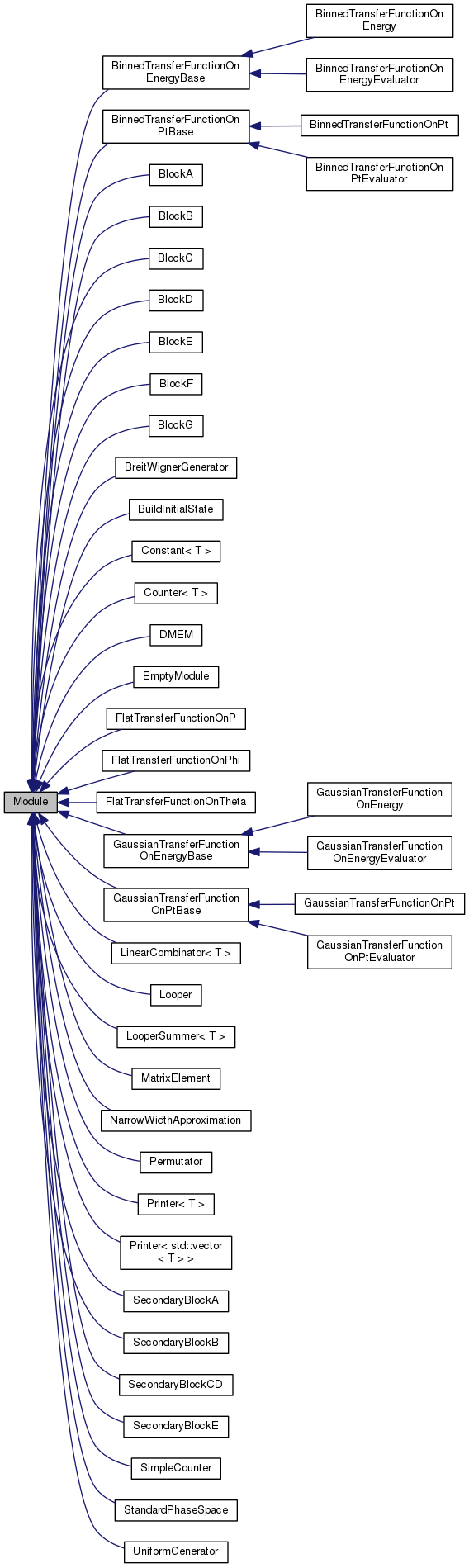
Classes | |
class | invalid_configuration |
Public Types | |
enum | Status : std::int8_t { OK, NEXT, ABORT } |
Public Member Functions | |
Module (PoolPtr pool, const std::string &name) | |
Constructor. More... | |
virtual void | configure () |
Called once at the beginning of the job. | |
virtual void | beginIntegration () |
Called once at the beginning of the integration. | |
virtual void | beginPoint () |
Called once when a new PS point is started. More... | |
virtual void | beginLoop () |
Called once at the beginning of a loop. More... | |
virtual Status | work () |
Main function. More... | |
virtual void | endLoop () |
Called once at the end of a loop. More... | |
virtual void | endPoint () |
Called once when a PS point is finished. More... | |
virtual void | endIntegration () |
Called once at the end of the integration. | |
virtual void | finish () |
Called once at the end of the job. | |
virtual std::string | name () const final |
Static Public Member Functions | |
static std::string | statusToString (const Status &status) |
static bool | is_virtual_module (const std::string &name) |
Test if a given name correspond to a virtual module. More... | |
Protected Member Functions | |
template<typename T , typename... Args> | |
std::shared_ptr< T > | produce (const std::string &name, Args... args) |
Add a new output to the module. More... | |
template<typename T > | |
Value< T > | get (const std::string &module, const std::string &name) |
template<typename T > | |
Value< T > | get (const InputTag &tag) |
Protected Attributes | |
PoolPtr | m_pool |
Constructor & Destructor Documentation
◆ Module()
|
inline |
Constructor.
This constructor is called when the module is instanciate. It's the only place where you can access the memory pool, so you must declare here all your module's inputs and outputs
- Parameters
-
pool The memory pool. Use this to grab the input you needs from other modules, and to declare your outputs name The name of this module.
Member Function Documentation
◆ beginLoop()
|
inlinevirtual |
Called once at the beginning of a loop.
Only relevant if the module is inside a loop
Reimplemented in SimpleCounter, and Counter< T >.
◆ beginPoint()
|
inlinevirtual |
Called once when a new PS point is started.
Only relevant if the module is inside a loop, since otherwise the module is called only once anyway
Reimplemented in Looper, LooperSummer< T >, and LooperSummer< T >.
◆ endLoop()
|
inlinevirtual |
◆ endPoint()
|
inlinevirtual |
◆ is_virtual_module()
|
inlinestatic |
◆ produce()
|
inlineprotected |
Add a new output to the module.
Call this function to add a new output to the module. This output will then be available to all the modules.
Example:
- Template Parameters
-
T The type of the output.
- Parameters
-
name The name of the output. Must be unique to this module. args Variable list of optional arguments. Will be passed to the constructor of T.
- Returns
- A std::shared_ptr<T> pointing to newly allocated memory in the memory pool. Change the content of this pointer to change the output.
◆ work()
|
inlinevirtual |
Main function.
This method is called for each integration step. The module's logic and work happen here.
You'll usually want to override this function if you want your module to perform some task.
Reimplemented in BinnedTransferFunctionOnEnergyEvaluator, MatrixElement, BinnedTransferFunctionOnPtEvaluator, GaussianTransferFunctionOnPtEvaluator, GaussianTransferFunctionOnEnergyEvaluator, BinnedTransferFunctionOnEnergy, BinnedTransferFunctionOnPt, Looper, BlockD, BlockF, BlockB, BlockE, BlockC, BlockG, GaussianTransferFunctionOnEnergy, GaussianTransferFunctionOnPt, BlockA, SecondaryBlockA, SimpleCounter, SecondaryBlockB, SecondaryBlockE, BuildInitialState, SecondaryBlockCD, BreitWignerGenerator, Permutator, LinearCombinator< T >, FlatTransferFunctionOnP, Printer< std::vector< T > >, FlatTransferFunctionOnPhi, FlatTransferFunctionOnTheta, UniformGenerator, StandardPhaseSpace, DMEM, Counter< T >, Printer< T >, LooperSummer< T >, and EmptyModule.
The documentation for this class was generated from the following files: