A class encapsulating a lua table. More...
#include <ParameterSet.h>
Detailed Description
A class encapsulating a lua table.
All the items inside the table will be converted to plain C++ type, and can be accessed via the ParameterSet::get() functions.
Only the following types are supported:
int64_t
double
bool
std::string
InputTag
ParameterSet
std::vector<>
of one of the above types
Consider the following lua snippet:
The resulting ParameterSet
will encapsulate three values:
x
of typedouble
=91.
y
of typestd::string
="string"
z
of typestd::vector<int64_t>
=[10, 20, 30, 40]
and you would use the following code to access the z
value:
- Todo:
- Document the freezing of the configuration in ConfigurationReader
Definition at line 82 of file ParameterSet.h.
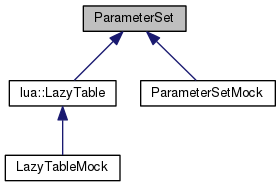
Classes | |
struct | Element |
A small wrapper around a momemta::any value. More... | |
Public Member Functions | |
template<typename T > | |
const T & | get (const std::string &name) const |
template<typename T > | |
T & | get (const std::string &name) |
template<typename T > | |
const T & | get (const std::string &name, const T &defaultValue) const |
const momemta::any & | rawGet (const std::string &name) const |
bool | exists (const std::string &name) const |
template<typename T > | |
bool | existsAs (const std::string &name) const |
template<typename T > | |
std::enable_if< std::is_same< T, bool >::value||std::is_same< T, InputTag >::value >::type | set (const std::string &name, const T &value) |
Change the value of a given parameter. If the parameter does not exist, it's first created. More... | |
template<typename T > | |
void | set (const std::string &name, const std::vector< T > &value) |
Change the value of a given parameter. If the parameter does not exist, it's first created. More... | |
template<typename T > | |
std::enable_if< is_string< T >::value >::type | set (const std::string &name, const T &value) |
Change the value of a given parameter. If the parameter does not exist, it's first created. More... | |
template<typename T > | |
std::enable_if< std::is_integral< T >::value &&!std::is_same< T, bool >::value >::type | set (const std::string &name, const T &value) |
Change the value of a given parameter. If the parameter does not exist, it's first created. More... | |
template<typename T > | |
std::enable_if< std::is_floating_point< T >::value >::type | set (const std::string &name, const T &value) |
Change the value of a given parameter. If the parameter does not exist, it's first created. More... | |
std::string | getModuleName () const |
std::string | getModuleType () const |
const ParameterSet & | globalParameters () const |
virtual ParameterSet * | clone () const |
Clone this ParameterSet. More... | |
std::vector< std::string > | getNames () const |
Protected Member Functions | |
ParameterSet (const std::string &module_type, const std::string &module_name) | |
virtual bool | lazy () const |
A flag indicating if this ParameterSet lazy loads its fields or not. More... | |
virtual void | create (const std::string &name, const momemta::any &value) |
Add a new element to the ParameterSet. More... | |
virtual void | setInternal (const std::string &name, Element &element, const momemta::any &value) |
virtual void | freeze () |
Protected Attributes | |
std::map< std::string, Element > | m_set |
Friends | |
class | ConfigurationReader |
class | Configuration |
class | ParameterSetParser |
class | momemta::ComputationGraph |
void | momemta::setInputTagsForInput (const momemta::ArgDef &, ParameterSet &, const std::vector< InputTag > &) |
Member Function Documentation
◆ clone()
|
virtual |
Clone this ParameterSet.
You must take ownership of the returned pointer.
- Returns
- A clone of this ParameterSet. You must take ownership of the returned value.
Reimplemented in lua::LazyTable.
Definition at line 97 of file ParameterSet.cc.
◆ create()
|
protectedvirtual |
Add a new element to the ParameterSet.
- Parameters
-
name Name of the new parameter value Value of the new parameter
Reimplemented in lua::LazyTable.
Definition at line 48 of file ParameterSet.cc.
◆ lazy()
|
protectedvirtual |
A flag indicating if this ParameterSet lazy loads its fields or not.
- Returns
- True if doing lazy loading, false otherwise
Reimplemented in lua::LazyTable.
Definition at line 39 of file ParameterSet.cc.
Referenced by ParameterSetParser::parse().
◆ rawGet()
const momemta::any & ParameterSet::rawGet | ( | const std::string & | name | ) | const |
Retrieve a raw value from this ParameterSet.
- Parameters
-
name The name of the parameter
- Returns
- The raw value of the parameter. A
not_found_error
exception is thrown ifparameter
does not exist in this set.
Definition at line 31 of file ParameterSet.cc.
Referenced by lua::inject_parameters().
◆ set() [1/5]
|
inline |
Change the value of a given parameter. If the parameter does not exist, it's first created.
- Parameters
-
name The name of the parameter to change value The new value of the parameter
Definition at line 160 of file ParameterSet.h.
Referenced by Configuration::getInputs().
◆ set() [2/5]
|
inline |
Change the value of a given parameter. If the parameter does not exist, it's first created.
Partial specialization for std::vector.
- Parameters
-
name The name of the parameter to change value The new value of the parameter
Definition at line 173 of file ParameterSet.h.
◆ set() [3/5]
|
inline |
Change the value of a given parameter. If the parameter does not exist, it's first created.
Specialization for string type, with implicit cast to std::string
- Parameters
-
name The name of the parameter to change value The new value of the parameter
Definition at line 194 of file ParameterSet.h.
◆ set() [4/5]
|
inline |
Change the value of a given parameter. If the parameter does not exist, it's first created.
Specialization for integral type, with implicit cast to int64_t
- Parameters
-
name The name of the parameter to change value The new value of the parameter
Definition at line 207 of file ParameterSet.h.
◆ set() [5/5]
|
inline |
Change the value of a given parameter. If the parameter does not exist, it's first created.
Specialization for floating-point type, with implicit cast to double
- Parameters
-
name The name of the parameter to change value The new value of the parameter
Definition at line 220 of file ParameterSet.h.
The documentation for this class was generated from the following files:
- include/momemta/ParameterSet.h
- core/src/ParameterSet.cc