Mathematical functions. More...
Detailed Description
Mathematical functions.
Definition in file Math.h.
#include <cmath>
#include <vector>
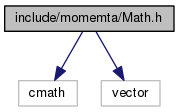

Go to the source code of this file.
Macros | |
#define | SQ(x) ((x) * (x)) |
Compute \( x^2 \). | |
#define | CB(x) ((x) * (x) * (x)) |
Compute \( x^3 \). | |
#define | QU(x) ((x) * (x) * (x) * (x)) |
Compute \( x^4 \). | |
Functions | |
template<typename T > | |
T | sign (const T &x) |
Sign function. More... | |
template<typename T > | |
double | dP_over_dE (const T &v) |
Compute \( \frac{dE}{dP} \). | |
double | jacobianNWA (const double mass, const double width) |
double | cosXpm2PI3 (const double x, const double pm) |
bool | solveQuadratic (const double a, const double b, const double c, std::vector< double > &roots, bool verbose=false) |
Finds the real solutions to \( a*x^2 + b*x + c = 0 \). More... | |
bool | solveCubic (const double a, const double b, const double c, const double d, std::vector< double > &roots, bool verbose=false) |
Finds the real solutions to \( a*x^3 + b*x^2 + c*x + d = 0 \). More... | |
bool | solveQuartic (const double a, const double b, const double c, const double d, const double e, std::vector< double > &roots, bool verbose=false) |
Finds the real solutions to \( a*x^4 + b*x^3 + c*x^2 + d*x + e = 0 \). More... | |
bool | solve2Quads (const double a20, const double a02, const double a11, const double a10, const double a01, const double a00, const double b20, const double b02, const double b11, const double b10, const double b01, const double b00, std::vector< double > &E1, std::vector< double > &E2, bool verbose=false) |
Solve a system of two quadratic equations. More... | |
bool | solve2QuadsDeg (const double a11, const double a10, const double a01, const double a00, const double b11, const double b10, const double b01, const double b00, std::vector< double > &E1, std::vector< double > &E2, bool verbose=false) |
Solve a system of two degenerated quadratic equations. More... | |
bool | solve2Linear (const double a10, const double a01, const double a00, const double b10, const double b01, const double b00, std::vector< double > &E1, std::vector< double > &E2, bool verbose=false) |
Solve a system of two linear equations. More... | |
double | BreitWigner (const double s, const double m, const double g) |
A relativist Breit-Wigner distribution. | |
bool | ApproxComparison (double value, double expected) |
Compare two doubles and return true if they are approximatively equal. | |
Function Documentation
◆ sign()
T sign | ( | const T & | x | ) |
Sign function.
- Returns
- 1 if
x
is positive, -1 ifx
is negative, or 0 if x is null
Definition at line 41 of file Math.h.
Referenced by solveQuadratic().
◆ solve2Linear()
bool solve2Linear | ( | const double | a10, |
const double | a01, | ||
const double | a00, | ||
const double | b10, | ||
const double | b01, | ||
const double | b00, | ||
std::vector< double > & | E1, | ||
std::vector< double > & | E2, | ||
bool | verbose = false |
||
) |
Solve a system of two linear equations.
Solves the system:
\[ \begin{align*} a_{10}E_1 + a_{01}E_2 + a_{00} &= 0 \\ b_{10}E_1 + b_{01}E_2 + b_{00} &= 0 \end{align*} \]
Appends the (x,y) solutions to the std::vectors E1, E2, making no attempt to check whether these vectors are empty.
Definition at line 357 of file Math.cc.
Referenced by solve2Quads(), and BlockA::work().
◆ solve2Quads()
bool solve2Quads | ( | const double | a20, |
const double | a02, | ||
const double | a11, | ||
const double | a10, | ||
const double | a01, | ||
const double | a00, | ||
const double | b20, | ||
const double | b02, | ||
const double | b11, | ||
const double | b10, | ||
const double | b01, | ||
const double | b00, | ||
std::vector< double > & | E1, | ||
std::vector< double > & | E2, | ||
bool | verbose = false |
||
) |
Solve a system of two quadratic equations.
Solves the system:
\[ \begin{align*} a_{20}E_1^2 + a_{02}E_2^2 + a_{11}E_1E_2 + a_{10}E_1 + a_{01}E_2 + a_{00} &= 0 \\ b_{20}E_1^2 + b_{02}E_2^2 + b_{11}E_1E_2 + b_{10}E_1 + b_{01}E_2 + b_{00} &= 0 \end{align*} \]
Which corresponds to finding the intersection points of two conics.
Appends the (x,y) solutions to the std::vectors E1, E2, making no attempt to check whether these vectors are empty.
In most cases it simply comes down to solving a quartic equation:
- eliminate the \( E_1^2 \) term
- solve for \( E_1 \) (linear!)
- inserting back gives a quartic function of \( E_2 \)
- find solutions for \( E_1 \)
The procedure becomes tricky in some special cases (intersections aligned along x- or y-axis, degenerate conics, ...)
Definition at line 192 of file Math.cc.
Referenced by SecondaryBlockE::work(), BlockC::work(), and BlockD::work().
◆ solve2QuadsDeg()
bool solve2QuadsDeg | ( | const double | a11, |
const double | a10, | ||
const double | a01, | ||
const double | a00, | ||
const double | b11, | ||
const double | b10, | ||
const double | b01, | ||
const double | b00, | ||
std::vector< double > & | E1, | ||
std::vector< double > & | E2, | ||
bool | verbose = false |
||
) |
Solve a system of two degenerated quadratic equations.
Solves the system:
\[ \begin{align*} a_{11}E_1E_2 + a_{10}E_1 + a_{01}E_2 + a_{00} &= 0 \\ b_{11}E_1E_2 + b_{10}E_1 + b_{01}E_2 + b_{00} &= 0 \end{align*} \]
Which corresponds to finding the intersection points of two conics.
Appends the (x,y) solutions to the std::vectors E1, E2, making no attempt to check whether these vectors are empty.
Referenced by solve2Quads().
◆ solveCubic()
bool solveCubic | ( | const double | a, |
const double | b, | ||
const double | c, | ||
const double | d, | ||
std::vector< double > & | roots, | ||
bool | verbose = false |
||
) |
Finds the real solutions to \( a*x^3 + b*x^2 + c*x + d = 0 \).
Handles special cases \( a = 0 \).
Appends the solutions to roots
, making no attempt to check whether the vector is empty.
- Note
- Multiple roots are present multiple times.
- Inspired by "Numerical Recipes" (Press, Teukolsky, Vetterling, Flannery), 2007 Cambridge University Press
- Parameters
-
a,b,c,d Coefficient of the equation [out] roots Roots of equation verbose If true, print the solution of the equation
- Returns
- True if a solution has been found, false otherwise
Definition at line 69 of file Math.cc.
Referenced by solveQuartic().
◆ solveQuadratic()
bool solveQuadratic | ( | const double | a, |
const double | b, | ||
const double | c, | ||
std::vector< double > & | roots, | ||
bool | verbose = false |
||
) |
Finds the real solutions to \( a*x^2 + b*x + c = 0 \).
Uses a numerically more stable way than the "classroom" method. Handles special cases \( a = 0 \) and / or \( b = 0 \). Appends the solutions to roots
, making no attempt to check whether the vector is empty.
- Note
- Double roots are present twice. See https://fr.wikipedia.org/wiki/Équation_du_second_degré::Calcul_numérique
- Parameters
-
a,b,c Coefficient of quadratic equation [out] roots Roots of equation verbose If true, print the solution of the equation
- Returns
- True if a solution has been found, false otherwise
Definition at line 26 of file Math.cc.
Referenced by solve2Quads(), solveCubic(), solveQuartic(), SecondaryBlockCD::work(), SecondaryBlockB::work(), SecondaryBlockA::work(), BlockE::work(), BlockB::work(), and BlockF::work().
◆ solveQuartic()
bool solveQuartic | ( | const double | a, |
const double | b, | ||
const double | c, | ||
const double | d, | ||
const double | e, | ||
std::vector< double > & | roots, | ||
bool | verbose = false |
||
) |
Finds the real solutions to \( a*x^4 + b*x^3 + c*x^2 + d*x + e = 0 \).
Handles special cases \( a = 0 \).
Appends the solutions to roots
, making no attempt to check whether the vector is empty.
The idea is to make a change of variable to eliminate the term in \( x^3 \), which gives a depressed quartic, then to try and factorize this quartic into two quadratic equations, which each then gives up to two roots. The factorization relies on solving a cubic equation (which is always possible), then taking the square root of one of these solution (ie there must be a positive solution)
- Note
- Multiple roots are present multiple times.
- See https://en.wikipedia.org/wiki/Quartic_function#Solving_by_factoring_into_quadratics and https://fr.wikipedia.org/wiki/Méthode_de_Descartes
- Parameters
-
a,b,c,d,e Coefficient of the equation [out] roots Roots of equation verbose If true, print the solution of the equation
- Returns
- True if a solution has been found, false otherwise
Definition at line 117 of file Math.cc.
Referenced by solve2Quads(), and BlockG::work().